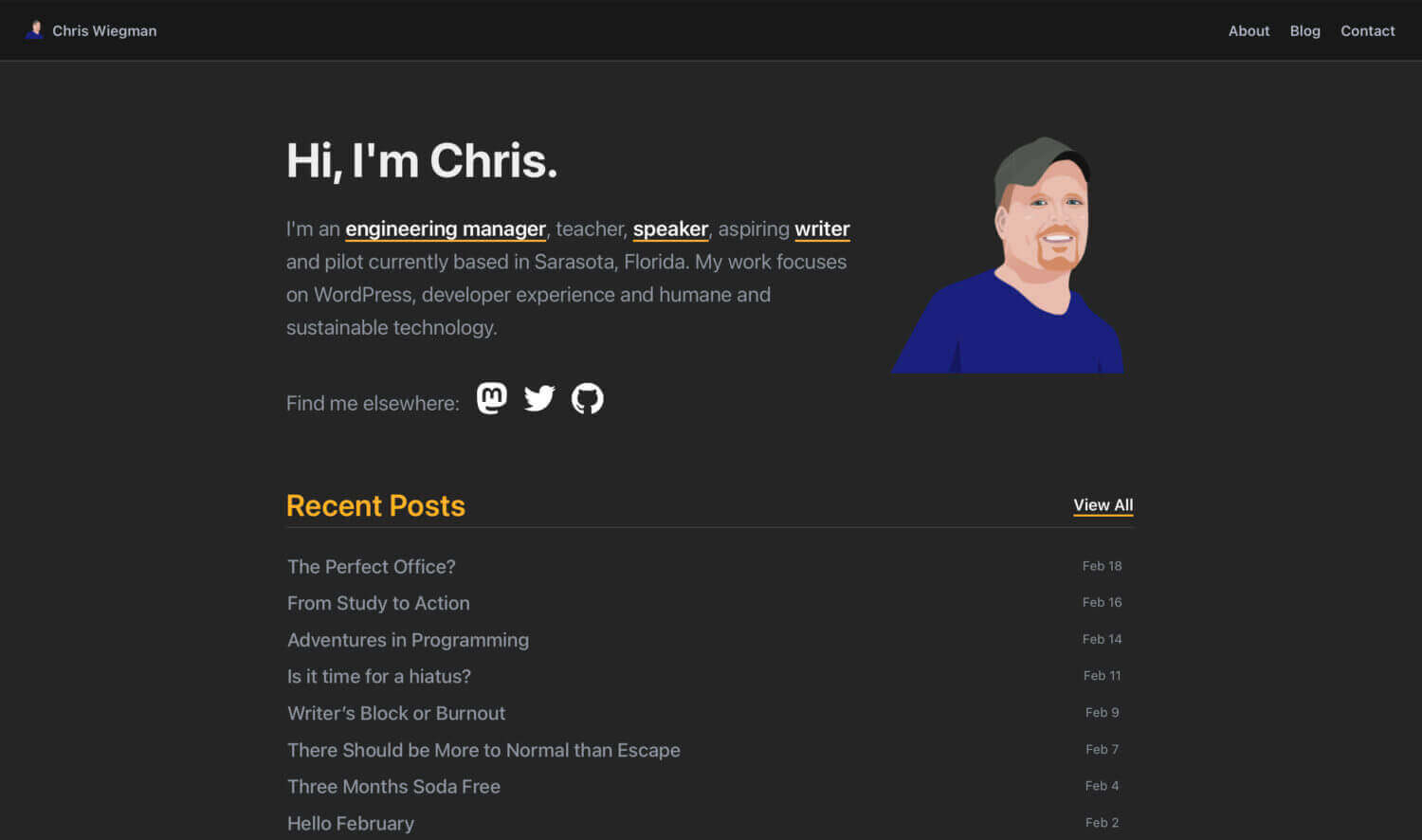
One of the features of this site is that the “blog” page is just that, a WordPress “page” instead of a formal index as the homepage is, according to WordPress, the official “latest posts” page in the Reading settings. While this setup works, the “blog” page itself only shows its last updated date as the date the page itself was saved. This is less than ideal for SEO and more as it appears I never update the “blog” page itself.
In addition to the blog page my “speaking” page is also built dynamically, this time from “event” and “talk” Custom Post Types created with The Pods Framework. This one is almost worse than the blog page not indicating it updated as there is no other home for the content on it.
How I updated the date on all the index pages
After brainstorming a few ideas to solve the problem I eventually realized that, at least for my simple use case, the best option was to simply update the post date on the pages themselves. This ensures a few things:
- The sitemap and other indexes are updated
- I can see that they’ve been updated myself in the WordPress Dashboard
- The pages I need to update are predictable making the cost of maintaining this relatively low
To implement this only requires a single hook, save_post, which gives us all we need to update the related page when an index item is updated. Here’s how I did it:
|
|
In my case this code lives in my functions.php file but it can go nearly anywhere. A few callouts with it:
First, you need to know the post IDs of the pages you want to update and associate them with the post types that should update them. You see this above in the post_types array.
Second, the checks for post type, wp_is_post_revision and post status are important. This ensures that the indexes are only updated when you actually click “update” or “publish” on the associated content.
Finally, removing the action ensures that we don’t risk calling any loop. This code will run once per save and that’s it.
Put together this code should be plenty to ensure you can use simple index pages for various content types and keep them current.